With the Simpplr client app created and configured we are ready to proceed forward with developing our custom app code needed to access APIs.
This process includes the following steps:
- Get the authorization code
- Use the authorization code to get an access token
- Use the access token to access the Simpplr API
- Use the refresh token to get the access token
Get the Authorization Code
The first step in the authentication process is to get an authorization code. To do this we need to hit a specific URL endpoint passing in a few parameters that are unique to your client application.
Those unique parameters are listed below:
Query param | Required | Value | Description |
---|---|---|---|
state | No | any string value | Include this in case you need to verify request. On successful authorization this will be included in your Redirect URL. |
scope | Yes | read or write or everything | read - in case you only want to access read resource. (GET API calls)write - in case you want to access write resource. (POST, PUT, DELETE API calls)everything - for both operations |
response_type | Yes | code | It should be code only. |
redirect_uri | Yes | Redirect URL used while creating application. | |
client_id | Yes | ClientId as received after creation of application. | |
access_type | No | offline | If this param is set to offline , you will receive refresh token in response.Refresh token is used to renew the access token. |
This process will involve following steps:
Redirecting to Authorize URL
You can create the authorization request using the OAuth Authorize URL that you received while creating the OAuth client application. For example - let's say the following are your OAuth application details:
clientID: 'client-id-unique'
clientSecret: 'client-secret-unique'
OAuth authorize URL: https://platform.app.simpplr.com/v1/identity/oauth/authorize
redirectURL: 'https://my-tenant.simpplr.xyz/success'
...then Authorization URL will be:
This URL either should directly be used in browser or via a button click in the UI to redirect browser to the authorize URL.
User Consent Page
Once you select Authorize URL, the user will be redirected to a page similar to the below image:
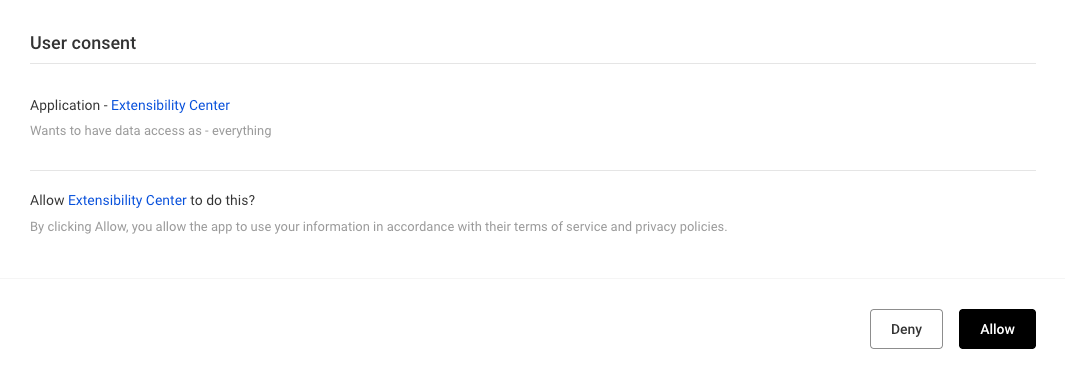
Please note: Your intranet users will be redirected to the tenant login page if not logged-in. After successfully logging in, they will be redirected to the above consent page.
“Allow” and “Deny“ will redirect users back to the provided redirect URL. However, “Deny“ will redirect with the error and “Allow“ will redirect to back with authorization code and state params (if provided).
For example: https://www.simpplr.com/callback?**code=b67e1958-40a2-4a74-b65b-dc9f3c182d6a**
The redirect URL will have the authorization code appended as query param named 'code'.
Getting an Access Token and Refresh Token
The authorization code generated at previous step can be exchanged with a token API to get the access token and refresh token. You must have received the token API URL while creating the OAuth client application.
Go through the Authorization API, this API will allow you to generate the access token and refresh token as per requirement.
Example curl request to this API:
curl --location 'https://platform.app.simpplr.com/v1/identity/oauth/token' \
--header 'Content-Type: application/json' \
--data '{
"grant_type": "authorization_code",
"auth_code": "auth-code-recievied",
"client_id": "client-id-unique",
"client_secret": "client-secret-unique"
}'
The access token received as response from this API can be used to call the Simpplr APIs.
Getting Access Token with Refresh Token
An access token is short lived - for offline access, it is advised to get the refresh token as well. Refresh token is used to get a new access token.
Authorization API allows you to re-new the access token. Example curl request for this:
curl --location 'https://platform.app.simpplr.com/v1/identity/oauth/token' \
--header 'Content-Type: application/json' \
--header 'Authorization: Bearer <RefreshToken>' \
--data '{
"grant_type": "refresh_token",
"client_id": "<clientId>",
"client_secret": "<clientSecret>"
}'
Refresh token will expire after 90 days of inactivity.